How to Disable Screen Orientation Change in Android?
Home - Blog Post
To disable screen orientation, and change in an Android app, you can set a fixed orientation for your entire application. Here are two common ways to achieve this:
Setting Fixed Orientation in AndroidManifest.xml:
You can specify the screen orientation in your app’s AndroidManifest.xml file by adding the android: screenorientation attribute to the activity element. This will lock the orientation for that specific activity.
For example, to set the orientation to portrait mode for a specific activity:
android:name=”.YourActivity”
android:screenOrientation=”portrait”>
<!– … other activity settings … –>
</activity>
Replace “portrait” with “landscape” if you want to lock it in landscape mode. If you want to allow any orientation changes, you can use “unspecified“.
Portrait Lock on Android
// add this in your androidManifest.xml (android/app/src/main/AndroidManifest.xml)
<activity android:name=”.MainActivity”
<…other code>
android:configChanges=”orientation” //<–ADD THIS
android:screenOrientation=”portrait”> //<–ADD THIS
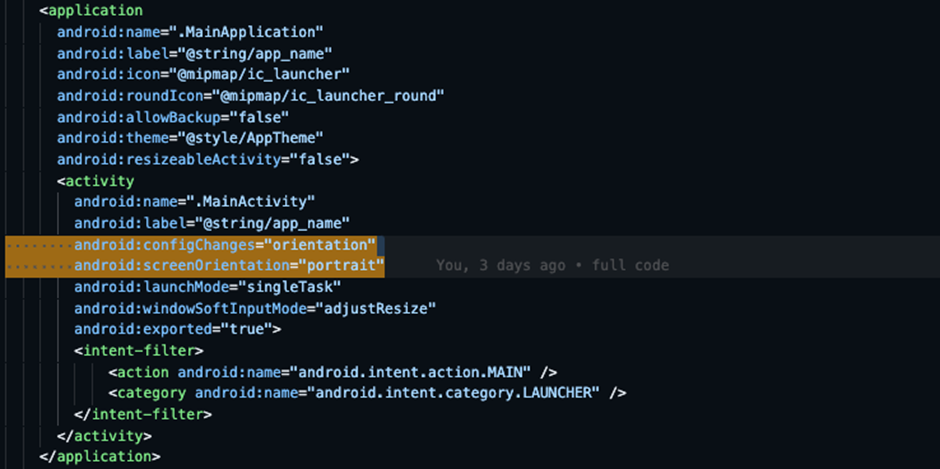
Setting Fixed Orientation Programmatically:
If you want to set the screen orientation programmatically at runtime, you can use the following Java code in your activity’s onCreate method:
For portrait mode:
setRequestedOrientation(ActivityInfo.SCREEN_ORIENTATION_PORTRAIT);
For landscape mode:
setRequestedOrientation(ActivityInfo.SCREEN_ORIENTATION_LANDSCAPE);
To allow any orientation changes, you can use
ActivityInfo.SCREEN_ORIENTATION_UNSPECIFIED.
For Kotlin, you can use the same code as above, just make sure it’s inside your activity’s onCreate method.
Disable Split Screen Android
//In your androidManifest.xml (android/app/src/main/AndroidManifest.xml)
<activity android:name=”.MainApplication” android:label=”@string/app_name” android:resizeableActivity=”false”//<<– ADD THIS
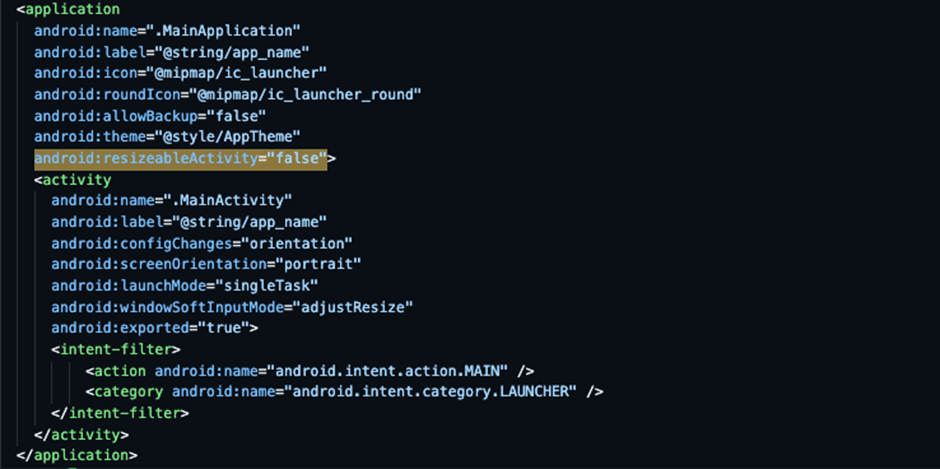
Set App Theme to Light in Android
// Changes in styles.xml (android/app/src/main/res/values/styles.xml)
<style name=”AppTheme” parent=”Theme.AppCompat.DayNight.NoActionBar”>
<!– Change DayNight to Light. –>
<style name=”AppTheme” parent=”Theme.AppCompat.Light.NoActionBar”>

By using either of these methods, you can effectively disable screen orientation changes for your Android application or specific activities within it. Choose the method that best suits your needs based on whether you want a fixed orientation for the entire app or just for specific activities.